基本数据类型的包装类java.lang.Integer是我们频繁使用的一个系统类,那么通过一个示例反应出的几个问题来深入理解一下此类的源码。
/** * 1.Integer时final的,表明其不能被继承 * 2.实现了implements Comparable<java.lang.Integer>接口说明其是可比较的 */public final class Integer extends Number implements Comparable<java.lang.Integer> { @Native public static final int MIN_VALUE = 0x80000000; @Native public static final int MAX_VALUE = 0x7fffffff; @SuppressWarnings("unchecked") public static final Class<java.lang.Integer> TYPE = (Class<java.lang.Integer>) Class.getPrimitiveClass("int"); final static char[] digits = { '0' , '1' , '2' , '3' , '4' , '5' , '6' , '7' , '8' , '9' , 'a' , 'b' , 'c' , 'd' , 'e' , 'f' , 'g' , 'h' , 'i' , 'j' , 'k' , 'l' , 'm' , 'n' , 'o' , 'p' , 'q' , 'r' , 's' , 't' , 'u' , 'v' , 'w' , 'x' , 'y' , 'z' }; public static String toString(int i, int radix) { if (radix < Character.MIN_RADIX || radix > Character.MAX_RADIX) radix = 10; /* Use the faster version */ if (radix == 10) { return toString(i); } char buf[] = new char[33]; boolean negative = (i < 0); int charPos = 32; if (!negative) { i = -i; } while (i <= -radix) { //直接根据i与进制radix的求余结果从digits[]里面取值,提高运算效率 buf[charPos--] = digits[-(i % radix)]; i = i / radix; } buf[charPos] = digits[-i]; if (negative) { buf[--charPos] = '-'; } return new String(buf, charPos, (33 - charPos)); } /** * @since 1.8 */ public static String toUnsignedString(int i, int radix) { return Long.toUnsignedString(toUnsignedLong(i), radix); } public static String toHexString(int i) { return toUnsignedString0(i, 4); } public static String toOctalString(int i) { return toUnsignedString0(i, 3); } public static String toBinaryString(int i) { return toUnsignedString0(i, 1); } /** * Convert the integer to an unsigned number. */ private static String toUnsignedString0(int val, int shift) { // assert shift > 0 && shift <=5 : "Illegal shift value"; int mag = java.lang.Integer.SIZE - java.lang.Integer.numberOfLeadingZeros(val); int chars = Math.max(((mag + (shift - 1)) / shift), 1); char[] buf = new char[chars]; formatUnsignedInt(val, shift, buf, 0, chars); // Use special constructor which takes over "buf". return new String(buf, true); } static int formatUnsignedInt(int val, int shift, char[] buf, int offset, int len) { int charPos = len; int radix = 1 << shift; int mask = radix - 1; do { buf[offset + --charPos] = java.lang.Integer.digits[val & mask]; val >>>= shift; } while (val != 0 && charPos > 0); return charPos; } final static char [] DigitTens = { '0', '0', '0', '0', '0', '0', '0', '0', '0', '0', '1', '1', '1', '1', '1', '1', '1', '1', '1', '1', '2', '2', '2', '2', '2', '2', '2', '2', '2', '2', '3', '3', '3', '3', '3', '3', '3', '3', '3', '3', '4', '4', '4', '4', '4', '4', '4', '4', '4', '4', '5', '5', '5', '5', '5', '5', '5', '5', '5', '5', '6', '6', '6', '6', '6', '6', '6', '6', '6', '6', '7', '7', '7', '7', '7', '7', '7', '7', '7', '7', '8', '8', '8', '8', '8', '8', '8', '8', '8', '8', '9', '9', '9', '9', '9', '9', '9', '9', '9', '9', } ; final static char [] DigitOnes = { '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', } ; /** * String中toString还是比较高效的 */ public static String toString(int i) { if (i == java.lang.Integer.MIN_VALUE) return "-2147483648"; int size = (i < 0) ? stringSize(-i) + 1 : stringSize(i); char[] buf = new char[size]; //将一个整数高效地逐位存入一个char数组中 getChars(i, size, buf); return new String(buf, true); } /** * @since 1.8 */ public static String toUnsignedString(int i) { return Long.toString(toUnsignedLong(i)); } static void getChars(int i, int index, char[] buf) { int q, r; int charPos = index; char sign = 0; if (i < 0) { sign = '-'; i = -i; } // Generate two digits per iteration while (i >= 65536) { q = i / 100; // really: r = i - (q * 100); r = i - ((q << 6) + (q << 5) + (q << 2)); i = q; buf [--charPos] = DigitOnes[r]; buf [--charPos] = DigitTens[r]; } // Fall thru to fast mode for smaller numbers // assert(i <= 65536, i); for (;;) { q = (i * 52429) >>> (16+3); r = i - ((q << 3) + (q << 1)); // r = i-(q*10) ... buf [--charPos] = digits [r]; i = q; if (i == 0) break; } if (sign != 0) { buf [--charPos] = sign; } } final static int [] sizeTable = { 9, 99, 999, 9999, 99999, 999999, 9999999, 99999999, 999999999, java.lang.Integer.MAX_VALUE }; /** * 内部使用 * 此判断某个数字的位数方法及其巧妙与高效,应值得借鉴 * 这样的话就避免了除法、求余等。效率应该及其高效。 */ static int stringSize(int x) { for (int i=0; ; i++) if (x <= sizeTable[i]) return i+1; } /** * 当String为null,会抛出null异常,注意返回的是int,不是Integer对象 */ public static int parseInt(String s, int radix) throws NumberFormatException { if (s == null) { throw new NumberFormatException("null"); } if (radix < Character.MIN_RADIX) { throw new NumberFormatException("radix " + radix + " less than Character.MIN_RADIX"); } if (radix > Character.MAX_RADIX) { throw new NumberFormatException("radix " + radix + " greater than Character.MAX_RADIX"); } int result = 0; boolean negative = false; int i = 0, len = s.length(); int limit = -java.lang.Integer.MAX_VALUE; int multmin; int digit; if (len > 0) { char firstChar = s.charAt(0); if (firstChar < '0') { // Possible leading "+" or "-" if (firstChar == '-') { negative = true; limit = java.lang.Integer.MIN_VALUE; } else if (firstChar != '+') throw NumberFormatException.forInputString(s); if (len == 1) // Cannot have lone "+" or "-" throw NumberFormatException.forInputString(s); i++; } multmin = limit / radix; while (i < len) { // Accumulating negatively avoids surprises near MAX_VALUE digit = Character.digit(s.charAt(i++),radix); if (digit < 0) { throw NumberFormatException.forInputString(s); } if (result < multmin) { throw NumberFormatException.forInputString(s); } result *= radix; if (result < limit + digit) { throw NumberFormatException.forInputString(s); } result -= digit; } } else { throw NumberFormatException.forInputString(s); } return negative ? result : -result; } public static int parseInt(String s) throws NumberFormatException { return parseInt(s,10); } public static int parseUnsignedInt(String s, int radix) throws NumberFormatException { if (s == null) { throw new NumberFormatException("null"); } int len = s.length(); if (len > 0) { char firstChar = s.charAt(0); if (firstChar == '-') { throw new NumberFormatException(String.format("Illegal leading minus sign " + "on unsigned string %s.", s)); } else { if (len <= 5 || // Integer.MAX_VALUE in Character.MAX_RADIX is 6 digits (radix == 10 && len <= 9) ) { // Integer.MAX_VALUE in base 10 is 10 digits return parseInt(s, radix); } else { long ell = Long.parseLong(s, radix); if ((ell & 0xffff_ffff_0000_0000L) == 0) { return (int) ell; } else { throw new NumberFormatException(String.format("String value %s exceeds " + "range of unsigned int.", s)); } } } } else { throw NumberFormatException.forInputString(s); } } public static int parseUnsignedInt(String s) throws NumberFormatException { return parseUnsignedInt(s, 10); } /** * valueOf(),默认是10进制有符号转换,原理也是parseInt, * 但是与parseInt不同得是,返回值类型为Integer */ public static java.lang.Integer valueOf(String s, int radix) throws NumberFormatException { return java.lang.Integer.valueOf(parseInt(s,radix)); } public static java.lang.Integer valueOf(String s) throws NumberFormatException { return java.lang.Integer.valueOf(parseInt(s, 10)); } /** * 静态内部类,里面包含了一个缓存数组cache[],默认情况下,[-128,127]之间的整数会在 * 第一次使用(类加载)被自动装箱,放在cache[]数组里。 */ private static class IntegerCache { static final int low = -128; static final int high; static final java.lang.Integer cache[]; static { // high value may be configured by property int h = 127; String integerCacheHighPropValue = sun.misc.VM.getSavedProperty("java.lang.Integer.IntegerCache.high"); if (integerCacheHighPropValue != null) { try { int i = parseInt(integerCacheHighPropValue); i = Math.max(i, 127); // Maximum array size is Integer.MAX_VALUE h = Math.min(i, java.lang.Integer.MAX_VALUE - (-low) -1); } catch( NumberFormatException nfe) { // If the property cannot be parsed into an int, ignore it. } } high = h; cache = new java.lang.Integer[(high - low) + 1]; int j = low; for(int k = 0; k < cache.length; k++) cache[k] = new java.lang.Integer(j++); // range [-128, 127] must be interned (JLS7 5.1.7) assert java.lang.Integer.IntegerCache.high >= 127; } private IntegerCache() {} } /** * 在这里会判断以下valueOf的数字大小,从而使用cache[]数组来构造. * 只是对于调用valueOf方法来构造的时候才会出现使用cache缓存的效果 * 而对于new Integer(100)的时候并不是 */ public static java.lang.Integer valueOf(int i) { if (i >= java.lang.Integer.IntegerCache.low && i <= java.lang.Integer.IntegerCache.high) return java.lang.Integer.IntegerCache.cache[i + (-java.lang.Integer.IntegerCache.low)]; return new java.lang.Integer(i); } /** * Integer得构造 */ private final int value; public Integer(int value) { this.value = value; } public Integer(String s) throws NumberFormatException { this.value = parseInt(s, 10); } /** * 将int转化为其他数字类型 * @return */ public byte byteValue() { return (byte)value; } public short shortValue() { return (short)value; } public int intValue() { return value; } public long longValue() { return (long)value; } public float floatValue() { return (float)value; } public double doubleValue() { return (double)value; } /** * 重写equals、hashCode、toString */ public String toString() { return toString(value); } @Override public int hashCode() { return java.lang.Integer.hashCode(value); } public static int hashCode(int value) { return value; } public boolean equals(Object obj) { if (obj instanceof java.lang.Integer) { return value == ((java.lang.Integer)obj).intValue(); } return false; } public static java.lang.Integer getInteger(String nm) { return getInteger(nm, null); } public static java.lang.Integer getInteger(String nm, int val) { java.lang.Integer result = getInteger(nm, null); return (result == null) ? java.lang.Integer.valueOf(val) : result; } public static java.lang.Integer getInteger(String nm, java.lang.Integer val) { String v = null; try { v = System.getProperty(nm); } catch (IllegalArgumentException | NullPointerException e) { } if (v != null) { try { return java.lang.Integer.decode(v); } catch (NumberFormatException e) { } } return val; } /** * 用于解析String得数值(二进制,八进制,是进制,十六进制) */ public static java.lang.Integer decode(String nm) throws NumberFormatException { int radix = 10; int index = 0; boolean negative = false; java.lang.Integer result; if (nm.length() == 0) throw new NumberFormatException("Zero length string"); char firstChar = nm.charAt(0); // Handle sign, if present if (firstChar == '-') { negative = true; index++; } else if (firstChar == '+') index++; // Handle radix specifier, if present if (nm.startsWith("0x", index) || nm.startsWith("0X", index)) { index += 2; radix = 16; } else if (nm.startsWith("#", index)) { index ++; radix = 16; } else if (nm.startsWith("0", index) && nm.length() > 1 + index) { index ++; radix = 8; } if (nm.startsWith("-", index) || nm.startsWith("+", index)) throw new NumberFormatException("Sign character in wrong position"); try { result = java.lang.Integer.valueOf(nm.substring(index), radix); result = negative ? java.lang.Integer.valueOf(-result.intValue()) : result; } catch (NumberFormatException e) { String constant = negative ? ("-" + nm.substring(index)) : nm.substring(index); result = java.lang.Integer.valueOf(constant, radix); } return result; } /** * 用于Integer或者int值进行大小比较 */ public int compareTo(java.lang.Integer anotherInteger) { return compare(this.value, anotherInteger.value); } /** * 此方法是java1.7新增的一个静态方法 * @since 1.7 */ public static int compare(int x, int y) { return (x < y) ? -1 : ((x == y) ? 0 : 1); } /** * @since 1.8 */ public static int compareUnsigned(int x, int y) { return compare(x + MIN_VALUE, y + MIN_VALUE); } /** * 以下这些方法都是Integer的一些内置运算方法 */ public static long toUnsignedLong(int x) { return ((long) x) & 0xffffffffL; } public static int divideUnsigned(int dividend, int divisor) { return (int)(toUnsignedLong(dividend) / toUnsignedLong(divisor)); } public static int remainderUnsigned(int dividend, int divisor) { // In lieu of tricky code, for now just use long arithmetic. return (int)(toUnsignedLong(dividend) % toUnsignedLong(divisor)); } @Native public static final int SIZE = 32; public static final int BYTES = SIZE / Byte.SIZE; public static int highestOneBit(int i) { // HD, Figure 3-1 i |= (i >> 1); i |= (i >> 2); i |= (i >> 4); i |= (i >> 8); i |= (i >> 16); return i - (i >>> 1); } public static int lowestOneBit(int i) { // HD, Section 2-1 return i & -i; } public static int numberOfLeadingZeros(int i) { // HD, Figure 5-6 if (i == 0) return 32; int n = 1; if (i >>> 16 == 0) { n += 16; i <<= 16; } if (i >>> 24 == 0) { n += 8; i <<= 8; } if (i >>> 28 == 0) { n += 4; i <<= 4; } if (i >>> 30 == 0) { n += 2; i <<= 2; } n -= i >>> 31; return n; } public static int numberOfTrailingZeros(int i) { // HD, Figure 5-14 int y; if (i == 0) return 32; int n = 31; y = i <<16; if (y != 0) { n = n -16; i = y; } y = i << 8; if (y != 0) { n = n - 8; i = y; } y = i << 4; if (y != 0) { n = n - 4; i = y; } y = i << 2; if (y != 0) { n = n - 2; i = y; } return n - ((i << 1) >>> 31); } public static int bitCount(int i) { i = i - ((i >>> 1) & 0x55555555); i = (i & 0x33333333) + ((i >>> 2) & 0x33333333); i = (i + (i >>> 4)) & 0x0f0f0f0f; i = i + (i >>> 8); i = i + (i >>> 16); return i & 0x3f; } public static int rotateLeft(int i, int distance) { return (i << distance) | (i >>> -distance); } public static int rotateRight(int i, int distance) { return (i >>> distance) | (i << -distance); } /** * 可以高效地将一个数字进行反转 */ public static int reverse(int i) { // HD, Figure 7-1 i = (i & 0x55555555) << 1 | (i >>> 1) & 0x55555555; i = (i & 0x33333333) << 2 | (i >>> 2) & 0x33333333; i = (i & 0x0f0f0f0f) << 4 | (i >>> 4) & 0x0f0f0f0f; i = (i << 24) | ((i & 0xff00) << 8) | ((i >>> 8) & 0xff00) | (i >>> 24); return i; } public static int signum(int i) { // HD, Section 2-7 return (i >> 31) | (-i >>> 31); } public static int reverseBytes(int i) { return ((i >>> 24) ) | ((i >> 8) & 0xFF00) | ((i << 8) & 0xFF0000) | ((i << 24)); } public static int sum(int a, int b) { return a + b; } public static int max(int a, int b) { return Math.max(a, b); } public static int min(int a, int b) { return Math.min(a, b); } //序列化 @Native private static final long serialVersionUID = 1360826667806852920L; }
原文链接:https://www.qiquanji.com/post/8446.html
本站声明:网站内容来源于网络,如有侵权,请联系我们,我们将及时处理。
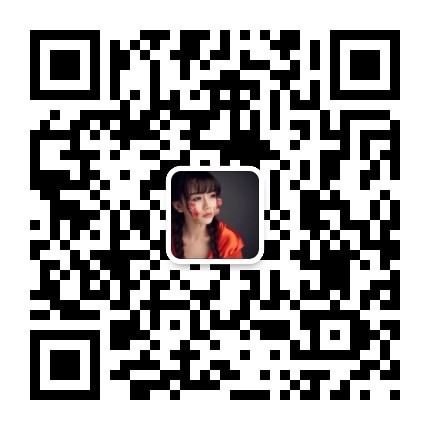
微信扫码关注
更新实时通知