基础知识
typedef struct cJSON { struct cJSON *next, *prev; struct cJSON *child; int type; char *valuestring; int valueint; double valuedouble; char *string; } cJSON;
解析/构建JSON数据
难度+
一层
{ "id" : "123", "version":"1.0", "method":"/sys/adsgag/test_device/thingrvice/propertyt" }
解析JSON
#include "stdio.h" #include "cJSON.h" typedef struct _DestStr { char* id; char* version; char* method; }DestStr; int decode_Json(char *src, DestStr* dest) { cJSON *root = cJSON_Parse(src); // 获取根目录 if (!root) { printf("get root faild !\n"); return -1; } cJSON *id = cJSON_GetObjectItem(root, "id"); if (!id) { printf("no id!\n"); return -1; } dest->id = malloc(strlen(id->valuestring) + 1); if (dest->id == NULL) { printf("error!!\n"); } strncpy(dest->id, id->valuestring, strlen(id->valuestring)); dest->id[strlen(id->valuestring)] = '\0'; cJSON *version = cJSON_GetObjectItem(root, "version"); if (!version) { printf("no version!\n"); return -1; } dest->version = malloc(strlen(version->valuestring) + 1); if (dest->version == NULL) { printf("error!!\n"); } strncpy(dest->version, version->valuestring, strlen(version->valuestring)); dest->version[strlen(version->valuestring)] = '\0'; cJSON *method = cJSON_GetObjectItem(root, "method"); if (!method) { printf("no method!\n"); return -1; } dest->method = malloc(strlen(method->valuestring) + 1); if (dest->method == NULL) { printf("error!!\n"); } strncpy(dest->method, method->valuestring, strlen(method->valuestring)); dest->method[strlen(method->valuestring)] = '\0'; if (root) cJSON_Delete(root); return 0; } int main(void) { DestStr* dest = (DestStr*)malloc(sizeof(DestStr)); char* srcStr = "{\n\"id\" : \"123\",\n\"version\" : \"1.0\",\n\"method\" : \"/sys/adsgag/test_device/thingrvice/propertyt\"\n}"; decode_Json(srcStr, dest); printf("dest->id:%s\n", dest->id); printf("dest->version:%s\n", dest->version); printf("dest->method:%s\n", dest->method); return 0; }
构建JSON
#include "stdio.h" #include "cJSON.h" char* encode_Json() // 构建 { cJSON * pJsonRoot = NULL; pJsonRoot = cJSON_CreateObject(); if (NULL == pJsonRoot) { //error happend here return NULL; } cJSON_AddStringToObject(pJsonRoot, "id", "123"); cJSON_AddStringToObject(pJsonRoot, "version", "1.0"); cJSON_AddStringToObject(pJsonRoot, "method", "/sys/adsgag/test_device/thingrvice/propertyt"); // cJSON_AddNumberToObject(pJsonRoot, "version", 10010); // cJSON_AddBoolToObject(pJsonRoot, "bool", 1); char * p = cJSON_Print(pJsonRoot); if (NULL == p) { cJSON_Delete(pJsonRoot); return NULL; } cJSON_Delete(pJsonRoot); return p; } int main(void) { printf("encode_Json:%s\n",encode_Json()); return 0; }
难度++
二层
{ "id" : "123", "version":"1.0", "params" : { "swtich" : 1, "usedTime": "50.5" }, "method":"/sys/adsgag/test_device/thingrvice/propertyt" }
解析JSON
#include "stdio.h" #include "cJSON.h" typedef struct _DestStr { char* id; char* version; char* method; int swtich; char* usedTime; }DestStr; int decode_Json(char *src, DestStr* dest) { cJSON *root = cJSON_Parse(src); // 获取根目录 if (!root) { printf("get root faild !\n"); return -1; } cJSON *id = cJSON_GetObjectItem(root, "id"); if (!id) { printf("no id!\n"); return -1; } dest->id = malloc(strlen(id->valuestring) + 1); if (dest->id == NULL) { printf("error!!\n"); } strncpy(dest->id, id->valuestring, strlen(id->valuestring)); dest->id[strlen(id->valuestring)] = '\0'; cJSON *version = cJSON_GetObjectItem(root, "version"); if (!version) { printf("no version!\n"); return -1; } dest->version = malloc(strlen(version->valuestring) + 1); if (dest->version == NULL) { printf("error!!\n"); } strncpy(dest->version, version->valuestring, strlen(version->valuestring)); dest->version[strlen(version->valuestring)] = '\0'; cJSON *method = cJSON_GetObjectItem(root, "method"); if (!method) { printf("no method!\n"); return -1; } dest->method = malloc(strlen(method->valuestring) + 1); if (dest->method == NULL) { printf("error!!\n"); } strncpy(dest->method, method->valuestring, strlen(method->valuestring)); dest->method[strlen(method->valuestring)] = '\0'; cJSON *params = cJSON_GetObjectItem(root, "params"); // 子节点 if (!params) { printf("no params!\n"); return -1; } cJSON *swtich = cJSON_GetObjectItem(params, "swtich"); // 从子节点里面找 if (!swtich) { printf("no swtich!\n"); return -1; } dest->swtich = swtich->valueint; cJSON *usedTime = cJSON_GetObjectItem(params, "usedTime"); if (!usedTime) { printf("no usedTime!\n"); return -1; } dest->usedTime = malloc(strlen(usedTime->valuestring) + 1); if (dest->usedTime == NULL) { printf("error!!\n"); } strncpy(dest->usedTime, usedTime->valuestring, strlen(usedTime->valuestring)); dest->usedTime[strlen(usedTime->valuestring)] = '\0'; if (root) cJSON_Delete(root); return 0; } int main(void) { DestStr* dest = (DestStr*)malloc(sizeof(DestStr)); char* srcStr = "{\n\"id\" : \"123\",\n\"version\" : \"1.0\",\n\"params\" : {\n\"swtich\" : 1,\n\"usedTime\" : \"50.5\"\n},\n\"method\" : \"/sys/adsgag/test_device/thingrvice/propertyt\"\n}"; decode_Json(srcStr, dest); printf("dest->id:%s\n", dest->id); printf("dest->version:%s\n", dest->version); printf("dest->method:%s\n", dest->method); printf("dest->swtich:%d\n", dest->swtich); printf("dest->usedTime:%s\n", dest->usedTime); return 0; }
构建JSON
#include "stdio.h" #include "cJSON.h" char* encode_Json() // 构建 { cJSON * pJsonRoot = NULL; // 创建父节点 pJsonRoot = cJSON_CreateObject(); if (NULL == pJsonRoot) { //error happend here return NULL; } cJSON_AddStringToObject(pJsonRoot, "id", "123"); cJSON_AddStringToObject(pJsonRoot, "version", "1.0"); cJSON_AddStringToObject(pJsonRoot, "method", "/sys/adsgag/test_device/thingrvice/propertyt"); // cJSON_AddNumberToObject(pJsonRoot, "version", 10010); // cJSON_AddBoolToObject(pJsonRoot, "bool", 1); cJSON * pSubJson = NULL; // 创建子节点 pSubJson = cJSON_CreateObject(); if (NULL == pSubJson) { // create object faild, exit cJSON_Delete(pJsonRoot); return NULL; } cJSON_AddNumberToObject(pSubJson, "swtich", 1); cJSON_AddNumberToObject(pSubJson, "useTime",50.5); cJSON_AddItemToObject(pJsonRoot, "params", pSubJson); // 子节点的“键”,子节点挂在父节点上 char * p = cJSON_Print(pJsonRoot); if (NULL == p) { cJSON_Delete(pJsonRoot); return NULL; } cJSON_Delete(pJsonRoot); return p; } int main(void) { printf("encode_Json:%s\n", encode_Json()); return 0; }
难度+++
三层
{ "id" : "123", "version":"1.0", "params" : { "RGB" : { "R":110, "G":2, "B":50 }, "swtich" : 1, "usedTime": "50.5" }, "method":"/sys/adsgag/test_device/thingrvice/propertyt" }
解析JSON
#include "stdio.h" #include "cJSON.h" typedef struct _DestStr { char* id; char* version; char* method; int swtich; char* usedTime; int R; int G; int B; }DestStr; int decode_Json(char *src, DestStr* dest) { cJSON *root = cJSON_Parse(src); // 获取根目录 if (!root) { printf("get root faild !\n"); return -1; } cJSON *id = cJSON_GetObjectItem(root, "id"); if (!id) { printf("no id!\n"); return -1; } dest->id = malloc(strlen(id->valuestring) + 1); if (dest->id == NULL) { printf("error!!\n"); } strncpy(dest->id, id->valuestring, strlen(id->valuestring)); dest->id[strlen(id->valuestring)] = '\0'; cJSON *version = cJSON_GetObjectItem(root, "version"); if (!version) { printf("no version!\n"); return -1; } dest->version = malloc(strlen(version->valuestring) + 1); if (dest->version == NULL) { printf("error!!\n"); } strncpy(dest->version, version->valuestring, strlen(version->valuestring)); dest->version[strlen(version->valuestring)] = '\0'; cJSON *method = cJSON_GetObjectItem(root, "method"); if (!method) { printf("no method!\n"); return -1; } dest->method = malloc(strlen(method->valuestring) + 1); if (dest->method == NULL) { printf("error!!\n"); } strncpy(dest->method, method->valuestring, strlen(method->valuestring)); dest->method[strlen(method->valuestring)] = '\0'; cJSON *params = cJSON_GetObjectItem(root, "params"); // 子节点 if (!params) { printf("no params!\n"); return -1; } cJSON *swtich = cJSON_GetObjectItem(params, "swtich"); // 从子节点里面找 if (!swtich) { printf("no swtich!\n"); return -1; } dest->swtich = swtich->valueint; cJSON *usedTime = cJSON_GetObjectItem(params, "usedTime"); if (!usedTime) { printf("no usedTime!\n"); return -1; } dest->usedTime = malloc(strlen(usedTime->valuestring) + 1); if (dest->usedTime == NULL) { printf("error!!\n"); } strncpy(dest->usedTime, usedTime->valuestring, strlen(usedTime->valuestring)); dest->usedTime[strlen(usedTime->valuestring)] = '\0'; cJSON* rgb = cJSON_GetObjectItem(params, "RGB"); // 孙节点 if (!rgb) { printf("no RGB!\n"); return -1; } cJSON* r_ = cJSON_GetObjectItem(rgb, "R"); if (!r_) { printf("no R!\n"); return -1; } dest->R = r_->valueint; cJSON* g_ = cJSON_GetObjectItem(rgb, "G"); if (!g_) { printf("no G!\n"); return -1; } dest->G = g_->valueint; cJSON* b_ = cJSON_GetObjectItem(rgb, "B"); if (!b_) { printf("no B!\n"); return -1; } dest->B = b_->valueint; if (root) cJSON_Delete(root); return 0; } int main(void) { DestStr* dest = (DestStr*)malloc(sizeof(DestStr)); char* srcStr = "{\n\"id\" : \"123\",\n\"version\" : \"1.0\",\n\"params\" : {\n\"RGB\" : {\n\"R\":110,\n\"G\" : 2,\n\"B\" : 50\n}, \n\"swtich\" : 1,\n\"usedTime\" : \"50.5\"\n}, \n\"method\":\"/sys/adsgag/test_device/thingrvice/propertyt\"\n}"; decode_Json(srcStr, dest); printf("dest->id:%s\n", dest->id); printf("dest->version:%s\n", dest->version); printf("dest->method:%s\n", dest->method); printf("dest->swtich:%d\n", dest->swtich); printf("dest->usedTime:%s\n", dest->usedTime); printf("dest->R:%d\n", dest->R); printf("dest->G:%d\n", dest->G); printf("dest->B:%d\n", dest->B); return 0; }
构建JSON
#include "stdio.h" #include "cJSON.h" char* encode_Json() // 构建 { cJSON * pJsonRoot = NULL; // 创建父节点 pJsonRoot = cJSON_CreateObject(); if (NULL == pJsonRoot) { //error happend here return NULL; } cJSON_AddStringToObject(pJsonRoot, "id", "123"); cJSON_AddStringToObject(pJsonRoot, "version", "1.0"); cJSON_AddStringToObject(pJsonRoot, "method", "/sys/adsgag/test_device/thingrvice/propertyt"); // cJSON_AddNumberToObject(pJsonRoot, "version", 10010); // cJSON_AddBoolToObject(pJsonRoot, "bool", 1); cJSON * pSubJson = NULL; // 创建子节点 pSubJson = cJSON_CreateObject(); if (NULL == pSubJson) { // create object faild, exit cJSON_Delete(pJsonRoot); return NULL; } cJSON_AddNumberToObject(pSubJson, "swtich", 1); cJSON_AddNumberToObject(pSubJson, "useTime",50.5); cJSON_AddItemToObject(pJsonRoot, "params", pSubJson); // 子节点的“键”,子节点挂在父节点上 cJSON * pSSubJson = NULL; // 创建孙节点 pSSubJson = cJSON_CreateObject(); if (NULL == pSSubJson) { // create object faild, exit cJSON_Delete(pJsonRoot); return NULL; } cJSON_AddNumberToObject(pSSubJson, "R", 110); cJSON_AddNumberToObject(pSSubJson, "G", 2); cJSON_AddNumberToObject(pSSubJson, "B", 50); cJSON_AddItemToObject(pSubJson, "RGB", pSSubJson); // 孙节点的“键”,孙节点挂在子节点上 char * p = cJSON_Print(pJsonRoot); if (NULL == p) { cJSON_Delete(pJsonRoot); return NULL; } cJSON_Delete(pJsonRoot); return p; } int main(void) { printf("encode_Json:%s\n", encode_Json()); return 0; }
原文:https://blog.csdn.net/qq_28877125/article/details/80047462?ops_request_misc=&request_id=&biz_id=102&utm_term=cjson%E4%BD%BF%E7%94%A8c%E8%AF%AD%E8%A8%80&utm_medium=distribute.pc_search_result.none-task-blog-2~all~sobaiduweb~default-3-80047462
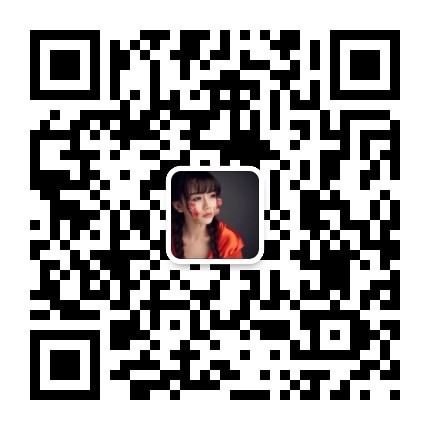
微信扫码关注
更新实时通知
评论列表: