#include "stm32f10x.h" #include "stdio.h" static GPIO_InitTypeDef GPIO_InitStructure; static NVIC_InitTypeDef NVIC_InitStructure; static USART_InitTypeDef USART_InitStructure; #define PB5OUT(n) *(volatile uint32_t *)(0x42000000+((uint32_t)&GPIOB->ODR-0x40000000)*32+n*4) //串口初始化 void led0(void){ //led0-->PB5 //使能端口 RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE); //初始化GPIO引脚 GPIO_InitStructure.GPIO_Pin=GPIO_Pin_5; GPIO_InitStructure.GPIO_Mode=GPIO_Mode_Out_PP; GPIO_InitStructure.GPIO_Speed= GPIO_Speed_50MHz;//高速响应 GPIO_Init(GPIOB, &GPIO_InitStructure); GPIO_SetBits(GPIOB,GPIO_Pin_5); } void delay_ms(uint32_t n){ while(n--){ SysTick->CTRL=0; //关闭系统定时器,第0位:使能位,1为使能 SysTick->LOAD=(72000)-1;//配置计数值,因为计算机从0开始,所以减一 SysTick->VAL=0;//清空当前的值(标志位),定时器在计数时,标志位为0,当计数完毕时,标志位为1 SysTick->CTRL=5;//5=101 使能系统计时器,并且使用系统时钟 //第二位:时钟选择位,0=系统时钟/8,1=系统时钟 while((SysTick->CTRL&0x10000)==0);//等待计数到0 //第16位:计数到0则为1 } SysTick->CTRL=0; } //printf函数重定向:修改fputc函数 int fputc(int c, FILE * stream){ USART_SendData(USART1, c); //判断有没有发送完毕 while(USART_GetFlagStatus(USART1,USART_FLAG_TXE)==RESET); return c; } void usart3_send_str(char *pstr){ char *p=pstr; while(*p!='\0'){ USART_SendData(USART3, *p); //判断有没有发送完毕 while(USART_GetFlagStatus(USART3,USART_FLAG_TXE)==RESET) ; p++; } } //At指令配置模块,蓝牙4.0模块不能跟手机进行连接 void ble_config_set(void){ //测试指令 usart3_send_str("AT\r\n"); delay_ms(500); //发送更改模块名字指令(需要复位生效,如果发送AT+RESET搜索蓝牙模块名字没有变更,那么请重新对蓝牙模块上电) usart3_send_str("AT+NAME小小\r\n"); delay_ms(500); usart3_send_str("AT+RESET\r\n"); delay_ms(500); } void usart3_init(uint32_t baud){ RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE); //使能PB10,PB11 RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART3, ENABLE); //配置txPB10 GPIO_InitStructure.GPIO_Pin=GPIO_Pin_10; GPIO_InitStructure.GPIO_Mode=GPIO_Mode_AF_PP; //设置成复用功能 GPIO_InitStructure.GPIO_Speed= GPIO_Speed_50MHz;//高速响应 GPIO_Init(GPIOB, &GPIO_InitStructure); //配置rxPB11 GPIO_InitStructure.GPIO_Pin=GPIO_Pin_11; GPIO_InitStructure.GPIO_Mode=GPIO_Mode_IN_FLOATING; //设置成复用功能 GPIO_Init(GPIOB, &GPIO_InitStructure); //配置串口 USART_InitStructure.USART_BaudRate = baud;//设置波特率 USART_InitStructure.USART_WordLength = USART_WordLength_8b;//8位数据位 USART_InitStructure.USART_StopBits = USART_StopBits_1;//1个停止位 USART_InitStructure.USART_Parity = USART_Parity_No;//无奇偶校验 USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;//无硬件数据流控制 USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;//接受和发送模式 USART_Init(USART3, &USART_InitStructure); USART_ITConfig(USART3, USART_IT_RXNE, ENABLE); //配置中断优先级 NVIC_InitStructure.NVIC_IRQChannel = USART3_IRQn; NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 3; NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0; NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2); NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE; NVIC_Init(&NVIC_InitStructure); //使能串口3 USART_Cmd(USART3, ENABLE); } void USART3_IRQHandler(void){ uint16_t xiao; //检测标志位 if(USART_GetITStatus(USART3, USART_IT_RXNE)==SET) { //接收数据 xiao=USART_ReceiveData(USART3); //将接收到的数据,返发给pc USART_SendData(USART1,xiao); while(USART_GetFlagStatus(USART1,USART_FLAG_TXE)==RESET); USART_ClearFlag(USART1,USART_FLAG_TXE); if(xiao=='1'){ PB5OUT(5)=0; }else{ PB5OUT(5)=1; } //清空标志位 USART_ClearITPendingBit(USART3,USART_IT_RXNE ); } } void usart1_init(uint32_t baud){ //串口PA9 ,PA10 //硬件时钟时钟打开 RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE); //使能串口1的时钟 RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE); //tx配置引脚的工作模式 GPIO_InitStructure.GPIO_Pin=GPIO_Pin_9; GPIO_InitStructure.GPIO_Mode=GPIO_Mode_AF_PP; //设置成复用功能 GPIO_InitStructure.GPIO_Speed= GPIO_Speed_50MHz;//高速响应 GPIO_Init(GPIOA, &GPIO_InitStructure); //USART1_RX GPIOA.10初始化 GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;//PA10 GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;//浮空输入 GPIO_Init(GPIOA, &GPIO_InitStructure);//初始化GPIOA.10 //配置串口 USART_InitStructure.USART_BaudRate = baud;//设置波特率 USART_InitStructure.USART_WordLength = USART_WordLength_8b;//8位数据位 USART_InitStructure.USART_StopBits = USART_StopBits_1;//1个停止位 USART_InitStructure.USART_Parity = USART_Parity_No;//无奇偶校验 USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;//无硬件数据流控制 USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;//接受和发送模式 USART_Init(USART1, &USART_InitStructure); //设置中断触发方式:接收一个字节触发中断 USART_ITConfig(USART1, USART_IT_RXNE, ENABLE); //配置中断优先级 NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn; NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 3; NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0; NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2); NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE; NVIC_Init(&NVIC_InitStructure); //使能串口1 USART_Cmd(USART1, ENABLE); } int main(){ usart1_init(115200); usart3_init(9600);//默认波特率 led0(); while(1){ printf("hhh"); delay_ms(3000); ble_config_set(); } }
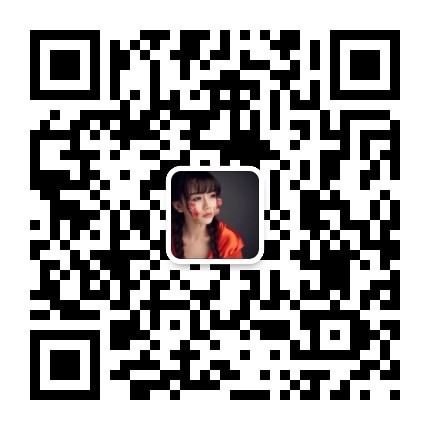
微信扫码关注
更新实时通知